Deferred Rendering is based around the idea that the rendering happens in multiple passes and the lighting calculations are deferred.
I have had only worked with forward rendering up until now. This is my process and experience with trying to execute deferred rendering with DirectX 11 and C++.
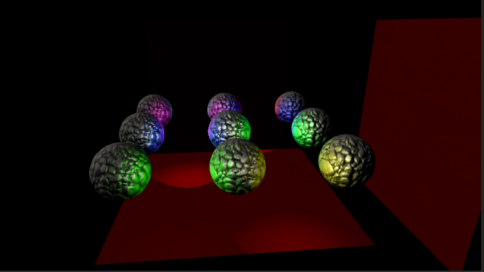
The first step is called the geometry pass, in which we render all the geometry information present in the scene. All of the information is rendered onto an array of textures which are required later in the lighting pass. The information that is saved in the 2D texture array is position vectors, normal vectors and color vectors. This texture array is called the G-Buffer (images below).
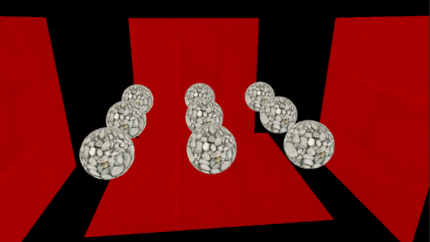
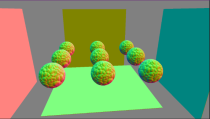
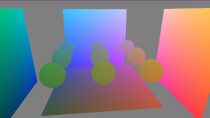
Setting up the G-buffer requires a Render Target array and a Shader Resource View array, to which the Pixel Shader renders. We can render to up to 8 Render targets, but we are only using 3 here.
The second step is the lighting pass. For each light, we render to a mesh with the corresponding shape of the light. For Directional light, we just use a quad, since it applies to all objects on the screen. For Point light, we use a sphere mesh and apply the lighting equation within the bounds of the sphere.
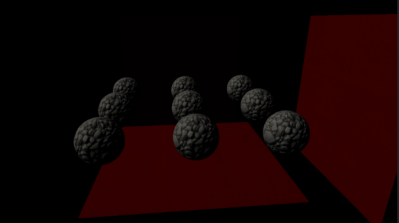
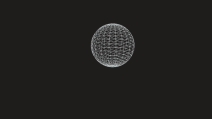
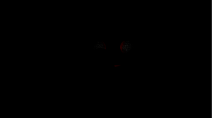
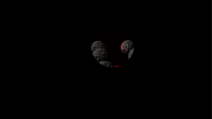
The pipeline for a single point light is shown here, the vertex shader outputs a sphere, the pixel shader runs the lighting equation within the given sphere mesh and the input assembler blends it with all the rest of the scene rendered till then.
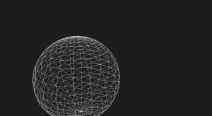
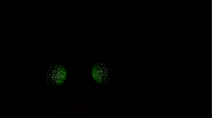
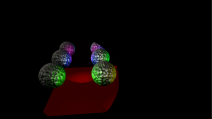
So we do this lighting pass for each light present in the scene and each light’s output is blended with the previous light’s output.
Some things to note before the lighting pass, the Blend state must be enabled with both the Source alpha and destination alpha at “one” and the blend operation set to “add”.
Also, the Rasterizer state must be enabled with the cull mode set to “cull back”.
Both the blend state and the rasterizer state must be disabled after the lighting pass so that they do not interfere with the rendering of the G-Buffer in the next frame.
Possible Optimizations/Updates :
- Add specular texture to the G-buffer
- Improve lighting calculation with multipass light rendering ( the lighting pass is done in multiple steps)
References :
- https://www.3dgep.com/forward-plus/#Deferred_Shading
- https://learnopengl.com/#!Advanced-Lighting/Deferred-Shading
- Chp. 11, Practical Rendering and Computation with Direct 3D by Zink, Pattineo & Hoxley
The code can be viewed on Github
Share this: